Once we have located the user (via a client or server
solution), we may want to display a map showing the user’s position,
and/or a list of points of interest or other information superposed on the
map.To do this, we should use one of the available public maps APIs:
Google Maps, Bing Maps from Microsoft, Yahoo! Maps, or OVI Maps from
Nokia. However, if we analyze mobile compatibility, there is really only
one choice: Google Maps. Compatibility for the others may increase in the
future, but at present Google’s API is by far the best supported.
There are actually two Google APIs that are useful for mobile
browsers: the Google Maps API v3 and the Google Maps Static API. The first
one is the same service that we can find in any website using Google Maps.
However, it is currently compatible only with iPhone and Android devices;
on other devices, this API will not work properly. The Static API will
allow us to show a static map compatible with any mobile browser.
Note:
Yahoo! APIs will be compatible if we use the Point of Interest
(POI) search or even the geocoding services. Microsoft also offers Bing
Maps Web Services with similar solutions.
1. Google Maps API v3
If we are sure that the device is an iPhone or Android
device, we should use the Google Maps API version 3, as shown in Figure 1.
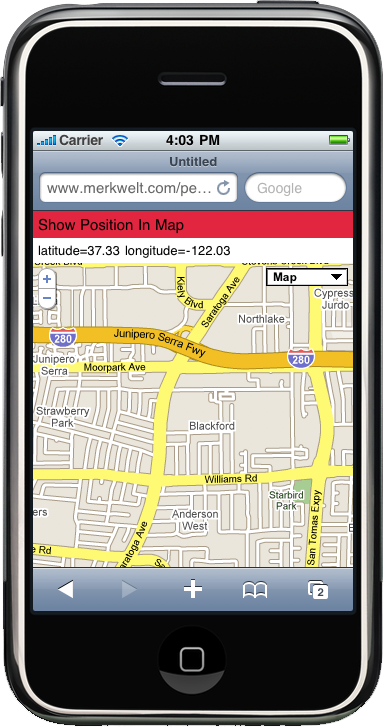
To use the API, we first need to include the script loader:
<script type="text/javascript"
src="http://maps.google.com/maps/api/js?sensor=true">
The sensor value must be
true if the device has geolocation
support via the operating system (this is the case on both iPhone and
Android devices). We should check whether the device is compatible
either on the server.
Then, we need to create a div
tag in our HTML and define its dimensions as 100%:
function showMap() {
var useragent = navigator.userAgent;
var divMap = document.getElementById("map");
if (useragent.indexOf('iPhone') != −1 || useragent.indexOf('Android') != −1 ||
useragent.indexOf(iPod) != −1 ) {
divMap.style.width = '100%';
divMap.style.height = '100%';
// ...
} else {
// Google Maps not compatible with this mobile device
}
}
Warning:
We should use the Google Maps API only for iPhone or Android
devices. On other devices, this will not work and we will need to use
the Google Maps Static API instead.
The other requirement for iPhone and Android from version 1.5 is
to define the meta tag to work
without user zooming and with an initial scale of 1.0. This is necessary to avoid usability
problems with the map zooming. So, in the head we should add:
<meta name="viewport" content="initial-scale=1.0, user-scalable=no" />
A full sample looks like this:
<html>
<head>
<meta name="viewport" content="initial-scale=1.0, user-scalable=no" />
<script type="text/javascript"
src="http://maps.google.com/maps/api/js?sensor=true"></script>
<script type="text/javascript">
function init() {
var useragent = navigator.userAgent;
var divMap = document.getElementById("map");
if (useragent.indexOf('iPhone') != −1 ||
useragent.indexOf('Android') != −1 ||
useragent.indexOf('iPod') != −1 ) {
divMap.style.width = '100%';
divMap.style.height = '100%';
position = getPosition(); // This needs to be implemented
var latlng = new google.maps.LatLng(position.latitude,
position.longitude);
var options = {
zoom: 7,
center: latlng,
mapTypeId: google.maps.MapTypeId.ROADMAP
};
var map = new google.maps.Map(document.getElementById("divMap"),
options);
} else {
// Google Maps not compatible with this mobile device
}
}
</script>
</head>
<body onload="init()">
<div id="divMap"></div>
</body>
</html>
To see the full Google Maps API documentation, go to http://code.google.com/apis/maps/documentation/v3.
2. Google Maps Static API
If we need compatibility with all mobile devices, even the
ones without JavaScript support, we can use the free Google Maps Static
API, which allows us to show a map as a static image without any
automatic interaction.
Warning:
To use this API you will need to sign up for a free API key at
http://code.google.com/apis/maps/signup.html.
This API is very simple and doesn’t require any JavaScript or
server code. We will use it inside an HTML image tag, in the source URL. The URL will
look like this:
http://maps.google.com/maps/api/staticmap?parameters
To use this API we need to get the location from the server or the
client and generate the image URL dynamically using JavaScript on
compatible devices. We need to remember that we may not want to show the
user’s location, but rather a map of some other place.
Note:
With the Google Maps Static API we can show a map on any mobile
phone on the market, even those without JavaScript or Ajax
support.
The common parameters include:
- sensor
Must be true for a mobile device.
- center
May be a position using
latitude,longitude,
or a city name.
- zoom
The level of zoom required, from 0 (world view) to 21 (building view).
- size
The size in pixels of the image required (e.g., 220×300). We should get the device’s
screen size from the server or from JavaScript.
- format
Accepts GIF, JPEG, or PNG. The default is PNG, which is suitable for mobile
devices.
- mobile
Marking this parameter as true creates different rendering images
optimized for viewing on mobile devices. Google suggests using
false in the case of iPhone or
Android devices.
Note:
The API is more complex, and it can even show marks and routes
over the map. To see the full documentation, go to http://code.google.com/apis/maps/documentation/staticmaps/.
For example, if we are using PHP and we just have the latitude and
longitude (acquired by any method), we should use:
<?php
// $latitude and $longitude already acquired
$url = "http://maps.google.com/maps/api/staticmap?center=$latitude,
$longitude&zoom=14&size=220x300&sensor=true&key=API_KEY";
?>
<img src="<?php echo $url ?>" width="220" height="300" />
3. Following LBS
Location-based services are great. We can create very
useful applications combining maps, places (Points of Interest), the
user’s location, and even other users’ locations. Some good books about
LBS and geographical services are available for marketers and
developers. I encourage you to look more deeply into this topic, so you
can create better mobile web experiences.