RGB decimal integer settings
A second way of mixing your colors using the rgb()
value assignment is to insert values from 0 to 255 (a total of 256
values because you count the 0), instead of the percentages used in the
earlier example. The value 256 represents the number of possible
combinations on two 8-bit bytes. In other words, it’s based on how a
computer stores and processes information. With a set of three values
from 0 to 255, you can generate 16,777,216 combinations. However, color
technology is far more complex than we can possibly discuss here, and
modern color processing keeps generating better color processors.
Suffice it to say, you can generate lots of colors with those
combinations of red, green, and blue. Here’s the format to assign a
color value:
rgb(
integerR,
integerG,
integerB);
For example, yellow, which mixes red and green would be
rgb(
255
,
255
,
0
);
It’s not as intuitive as HSL, but after a while,
you start getting a sense of mixes based on 256 values rather than
percentages. The following example shows a simple implementation.
<!
DOCTYPE HTML>
<
html>
<
head>
<
style type=
”text/css”
>
body {
/* Red background */
background-
color:
rgb(
255
,
0
,
0
);
}
h1 {
/* Big Yellow Text */
color:
rgb(
255
,
255
,
0
);
font-
family:
”Arial Black”
,
Gadget,
sans-
serif;
}
h2 {
/*Blue Text + Gray Background */
color:
rgb(
0
,
0
,
255
);
background-
color:
rgb(
150
,
150
,
150
);
}
</
style>
<
meta http-
equiv=
”Content-Type”
content=
”text/html; charset=UTF-8”
>
<
title>
Decimal Colors</
title>
</
head>
<
body>
<
h1>&
nbsp;
Big Yellow Header</
h1>
<
h2>&
nbsp;
Blue header with a gray background</
h2>
</
body>
</
html>
The only difference between using RGB with values
from 0 to 255 and 0 percent to 100 percent is in perception. You may be
thinking that you can be more precise with your colors using the 256
values instead of the 0-to-100 range of percentages, but that isn’t the
case because you can use fractions in percentage assignments. Whether
you use the percentage notation or the 0-to-255 notation really comes
down to a matter of personal preference. Figure 4-4 shows the outcome
using the Opera Mini browser on an iPhone.
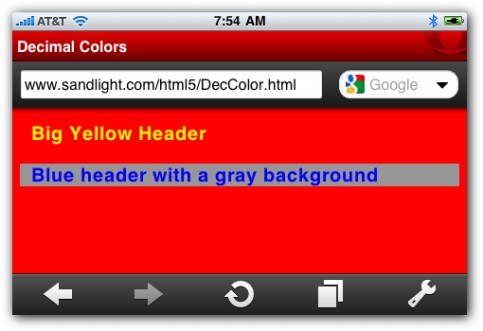
Figure 4: Colors mixed using integer values, shown on a mobile device.
As you can see in Figure 4, the mobile device is
not picking up the Arial Black font, but it has no problems with the
colors. Be sure to check your mobile device for fonts and other effects
if they’re essential to how your page looks. Remember:
Most computers have a far more complete set of fonts and styles than
mobile devices do. In time, though, they should be very similar.
Hexadecimal settings: Thinking like your computer
The value 6F001C
generates a rich mocha red. If
we break it down, we can see that it, too, is simply a mixture of red,
green, and blue. But to understand what’s going on, we need to
understand a little about computer numbering systems.
We’re used to counting using a decimal system. We
use the values 0 through 9 (ten digits), and once those ten digits are
used up we start over with two digits — 1 and 0 — which we call “ten.”
As you may know, computers are based on switches being in an On state
or an Off state. By substituting a “1” for On and a “0” for Off, we can
write a code based on a binary system using 1s and 0s; so instead of
having ten digits to work with, we have only two. Table 4.2 shows what
it takes to count up to 16 using the binary system. It also includes a
third column that shows a base-16 numbering system called hexadecimal.
Table 4.2 Numbering Systems
|
Binary
|
Decimal
|
Hexadecimal
|
0
|
0
|
0
|
1
|
1
|
1
|
10
|
2
|
2
|
11
|
3
|
3
|
100
|
4
|
4
|
101
|
5
|
5
|
110
|
6
|
6
|
111
|
7
|
7
|
1000
|
8
|
8
|
1001
|
9
|
9
|
1010
|
10
|
A
|
1011
|
11
|
B
|
1100
|
12
|
C
|
1101
|
13
|
D
|
1110
|
14
|
E
|
1111
|
15
|
F
|
Each of the binary values is called a bit. A group
of bits is called a byte. In Table 4.2, the largest binary value is a
4-bit byte. Computers are arranged in different types of bytes, and the
8-bit byte is commonly used as a general reference to a byte. However,
modern computers are actually organized into 8-, 16-, 32-, 64-, and
even 128-bit bytes. (They just keep getting bigger, so don’t expect
128-bit bytes to be the top limit.)
The highest value for a binary counting system in
an 8-bit byte is 11111111. When you look at that compared with decimal
and hexadecimal numbers, you see a very interesting pattern, as shown
in Table 4.3.
Table 4.3 Byte Values
|
Binary
|
Decimal
|
Hexidecimal
|
11111111
|
255
|
FF
|
As you can see in Table 4-3, the hexadecimal value
FF is the highest possible value for two digits; similarly, the binary
value 11111111 is the highest possible value for eight digits (a byte).
However, the decimal number is three digits and does not represent a
limit for those digits. In other words, the decimal system isn’t very
symmetrical with the binary counting system, but the hexadecimal system
is.
As you know, the RGB system of assigning integers
to color values uses values from 0 to 255. Using hexadecimal values,
you need only two digits (actually, hexadecimal integers) to represent
all 256 values in an 8-bit byte. It’s neater.
This leads to using hexadecimal integers in
assigning color values. Using six values — two each for red, green, and
blue — all the color values can be assigned using six hex integers. So
returning to the value 6F001C
, we can see the following:
Red: 6F
Blue: 00
Green: 1C
Getting used to hexadecimal can take some time, but
once you do, it’s easy to add color values with them. Also, you can
understand them in the same way as RGB decimal integers, but instead of
values of 0 to 255, you use 00 to FF. The following example shows some color using hexadecimals.
<!
DOCTYPE HTML>
<
html>
<
head>
<
style type=
”text/css”
>
/* Palette -- only use these colors!
69675C, 69623D, ECE8CF, E8D986, B5AA69
gray, olive, cream, dark cream, khaki */
body {
font-
family:
”Comic Sans MS”
,
cursive;
background-
color:
#ECE8CF;
color:
#69675C;
}
h1 {
font-
family:
”Arial Black”
,
Gadget,
sans-
serif;
color:
#B5aa60;
background-
color:
#E8D986;
text-
align:
center;
}
h2 {
font-
family:
”Lucida Sans Unicode”
,
“Lucida Grande”
,
sans-
serif;
color:
#b5aa69;
}
</
style>
<
meta http-
equiv=
”Content-Type”
content=
”text/html; charset=UTF-8”
>
<
title>
Hexadecimal with Palette</
title>
</
head>
<
body>
<
h1>
Style with a Color Palette</
h1>
<
h2>&
nbsp;
Desert in the Fall</
h2>
In the fall,
when the air cools a bit,
the desert begins to settle down and cloak itself in a warmer set of hues.
</
body>
</
html>
This example uses a color palette and simply places the color values in a comment within the <style>
container so that it can be viewed while putting the Web page together. Figure 5 shows what you can expect to see.
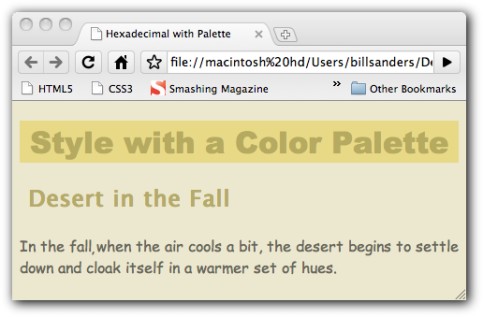
Figure 5: A hexadecimal color palette.
The colors belong to a set of colors that create a
certain mood or feeling. This one, “Desert in the Fall” was based on
what the designer believed to be a palette representing that time of
year in the desert.